Welcome to tutorial part 7. In this tutorial, we are going to learn about static files in Django.
What are the static files in Django?
Static files in Django are basically a folder that we create to store the images, CSS, javascript files in order to display them to the client in the browser and serve the client.
This will separate Python code from other codes and prevent the disorder in our project. Because dealing with the numerous sets of static files offered by each program becomes difficult in larger projects – especially those involving multiple apps or multiple images like online shoppings which there should be several photos for each item on the store.
In the previous tutorial, we have rendered a basic template. In this tutorial, we want to show how to serve static files in Django to the client.
But in Django, it’s not a simple as saying ok, in this HTML template I want to show an image with an img tag with an image address that is saved in some kind of folder inside the project. We have to set explicitly up static inside our Django project.
when we create a website for production we wouldn’t let Django typically serve up our images because at best it’s inefficient. we would use other services like AWS or Google cloud. But in local development, we will let Django takes care of static files. In order to let Django do that we need to do several steps and configuration.
Static files in settings.py
Open the settings.py and inside the installed app check django.contrib.staticfiles.
Django provides django.contrib.staticfiles built-in app to help manage static files.
This django. contrib. staticfiles app aggregates static files from all of your applications or any other locations you set out into a single location that can be served in production with ease.
This section does not require any revision or changes. Leave it as it is.
Configuring satatic URL in settings.py
In settings.py as you can see we have a static URL by default in our project settings.
STATIC_URL = '/ static/'
This is the address that we tell Django you have to go to find our static files. For example, if we have file index.css file on our website(let’s say doprax.com) the address of this file would be like this:
doprax.com/static/index.css
Also, we change this URL to anything we want for example:
STATIC_URL = '/ assest/'
Then the address would be doprax.com/assest/index.css. It still works but it’s best practice to keep it static.
We need to add another setting because Django does not know where exactly our static files are in our projects and where we store them.
the way to that is to add another property to settings as follows:
STATICFILES_DIRS = ( os.path.join(BASE_DIR,'assets'), )
It’s a tuple and should be in the capital. BASE_DIR is an acronym for the base directory which is built-in Django and grabs the base directory which is the same address as our main project directory. The string would be a directory inside the base project where we store our static files.
Almost in every case, It’s assets but it’s up to you to call it whatever you want.
So this STATICFILES_DIRS property tells that static files are gonna be found inside the static directory in the base directory.
Let’s save this and create an assets folder inside the base directory.
proj1 mkdir assets
We can save and store all our static files in this folder. Let’s create a CSS file and include it in basic_html.html which we already have to give our template a little styling.
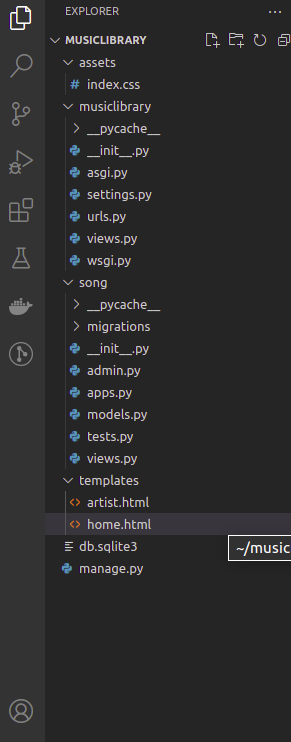
In this CSS file we added a blue background for the whole page then include it in our HTML template:
<link rel="stylesheet" href="/static/index.css/">
We can also include our CSS file dynamically. First, you need to load static in the head tag like this:
<head> {% load static %} <title>HTML basics</title> <link rel="stylesheet" href="{% static 'index.css' %}"> </head>
This is a much better way of loading static files because if we want to change the static_url for any reason, we don’t have to go through all the project’s code and change each one of the addresses accordingly.
Set Up static in urls.py
For setting up static files in URLs, open urls.py and import this:
from django.contrib.staticfiles.urls import staticfiles_urlpatterns from django.conf.urls.static import static
This is built-in package lets us append to URL patterns so that Django can handle serving static files and the way we do that is by adding this line of code:
urlpatterns+=staticfiles_urlpatterns()
If you wish to join a community of self-starters learning new skills and creating projects from scratch, join our Discord channel here and share your passion.
This is how we add and configure static files in Django. I hope you find it useful. See you in the next tutorial. 🙂
NEXT:
Django Tutorial for beginners [step by step] part 8 – User authentication Django
PREVIOUS: