Template in Django
Hello fellow developers, welcome to tutorial part 2. In this tutorial, we’re going to talk about templates in Django and also review some basic HTML and make a simple home page for our website.
Before creating a template file, It’s important to have a little perspective on the backend and frontend of a web application. When we talk about the Frontend of a website, we mean the parts that are visible on the browser, and users can interact with them. Everything you see on a website is a collection of HTML, CSS, and JavaScript that are all managed by your web browser. Items like pages, menus, buttons, fonts, contact forms and…, etc.
Django is for the most part a backend framework. A “backend” generally consists of three parts: a server, an application, and a database. When you submit a form, the data you enter travels to a server somewhere on the internet and is processed there and the necessary processes like charging your credit card and issuing a ticket will run. Django’s solution for the front-end of a website is HTML templates.
Setup Django templates
Create a folder in the root of your Django project and name it templates. Open a terminal go to the root of your project and
mkdir templates
In the previous tutorial, I briefly mentioned the setting.py file inside the main project folder(“~/musiclibrary/musiclibrary/settings.py”). Now open this file in your editor of choice and find the section about templates and add 'DIRS': ['templates'],
to DIRS
which is the second item in the dictionary below. In the end, it should look like this
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': ['templates'],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
Here we told Django to look for templates file in a directory named templates in the BASE_DIR which as the name suggests is the root of our Django project.
Now it is time to create add some HTML to our simple project. Create a file in the templates directory and name it home.html. In the next few sections, we will review HTML basics and add some HTML code to the home.html file.
What is HTML
HTML stands for HyperText Markup Language. HTML technically is not a programming language, but a markup language. HTML helps you define the overall structure and structure of your web page(frontend) using tags and where the menu, footer, header, and form should be. For example, you want to build a house. The first step is to build the skeleton and overall shape of the building using cement, concrete, rebar, and so on. In fact, first, you build the overall structure of the building, and then, using paint and plaster to design it. Web design with HTML is almost the same.
HTML tags
HTML tags are markups. Each of these tags has a specific meaning and has features such as changing the appearance of texts, creating different lists, and linking pages. The type of tags in HTML is diverse and constantly improving in new versions. Some of them are not very popular and are rarely used by web designers. For example, the meter tag, which is for measurement, is rarely used by site designers.
The first sentence of each HTML document is <! DOCTYPE html>. This section actually tells you this is an HTML page. If you take a look at the template we created, you will see the <! DOCTYPE html> in the first line. So keep in mind that we always put it in the first line of our code. The second main element of any HTML page is <html> and <html /> tags, which we use to start writing HTML. From now on, all the contents of an HTML page will be written between these two tags. Tags are elements that have different functions. HTML uses tags to place different elements and each user uses them according to what they want to create.
The head and body tags are among the main HTML tags that include sub-tags, elements, and internal content of a web page.
The title tag is required to display the page title in the browser, this is the text that displays in the browser tab for each page.
<head> <title>Home</title> </head>
The h1 tag indicates the headers that can have values up to h6, the larger the numerical values in front of h, the less important the text inside the tag, for example, the h1 tag is more important than the h3 tag!
<h1>Music library</h1> <h2>Rock music</h2> <h3>Famous Artist: </h3> <h4>Jimi Hendrix</h4> <h4>Chuck berry</h4> <h4>Eric Clapton</h4> <h2>wikipedia: </h2>
The p tag in HTML code indicates a paragraph, we said that HTML is a markup language and p in this markup represents a paragraph.
<body> <p>Music library is a place where you can find the information about the most beautiful songs from your favourite artists! enjoy!</p> </body>
The ul tag in HTML codes shows a list. each item in this tag starts with <li> tags.
<ul> <li>Bass guitar</li> <li>electric guitar</li> <li>Electronic drum</li> </ul>
The <a> tag is for the link referencing, as you can see the in code component, by this tag we have referenced the music platforms.
<a href="https://en.wikipedia.org/wiki/Rock_music">Rock music </a>
The <img> tag is for the images. This tag, unlike the other tags, doesn’t have an ending part.
<img src="https://upload.wikimedia.org/wikipedia/commons/thumb/0/02/Chuck_Berry_circa_1958.jpg/170px-Chuck_Berry_circa_1958.jpg">
All of the HTML codes above in a single HTML file are like this:
<!DOCTYPE html> <html> <body> <head> <title>Home</title> {% load static %} <link rel="stylesheet" href="{% static 'index.css' %}"> </head> <h1>Music library</h1> <p>Music library is a place where you can find the information about the most beautiful songs from your favourite artists! enjoy!</p> <h2>Rock music</h2> <p>Rock music is a broad genre of popular music that originated as "rock and roll" in the United States in the late 1940s and early 1950s, developing into a range of different styles in the mid-1960s and later, particularly in the United States and the United Kingdom</p> <img src="https://upload.wikimedia.org/wikipedia/commons/thumb/0/02/Chuck_Berry_circa_1958.jpg/170px-Chuck_Berry_circa_1958.jpg"> <h3>Famous Artist: </h3> <h4>Jimi Hendrix</h4> <h4>Chuck berry</h4> <h4>Eric Clapton</h4> <h2>wikipedia: </h2> <a href="https://en.wikipedia.org/wiki/Rock_music">Rock music </a> <h3>Typical instruments </h3> <ul> <li>Bass guitar</li> <li>electric guitar</li> <li>Electronic drum</li> </ul> <h3>Cultural origins</h3> <p>1950s and 1960s, US and UK</p> </body> </html>
Render
In order to easily return an HTML to our user, Django provides us a function called render, We call this function and feed it the HTML templates and also data and it goes ahead and basically fills it like a form and returns the result as an HTML response. More on this later. In our main app which is the same name as our project, it usually doesn’t have views.py file. you can create views.py and open it and add the following code
def home(request):
return render(request,"home.html")
With these two lines of code, we have defined a view function that handles user requests and essentially returns the HTML template that we have created (“~/musiclibrary/templates/basic_html.html”)
Now we want to know what does our page look like. But first, we need to create a way for users to reach this page. We do that by creating a URL entry in the urls.py file (“~/musiclibrary/musiclibrary/urls.py”). the URL patterns of the urls.py file should look something like this.
urlpatterns = [
path('admin/', admin.site.urls),
path('say-hello/', views.hello_world),
path('', views.home),
here we are telling Django that if a request arrives at “/” it should be handled by the home function that we have created above.
How it looks like in the browser
Here is a screenshot of the HTML codes we wrote together in the browser. If you’re thinking of becoming a more serious web developer regardless of backend or frontend development, learning HTML and CSS makes it easier for you to start since HTML is very easy to learn, and as a result continuing the journey of web development wouldn’t be exhausting and boring. It is one of the basic and essential languages in programming. The page that we have created may seem very primitive for now but you will be surprised when you see folks creating stunning websites using these simple blocks of HTML.
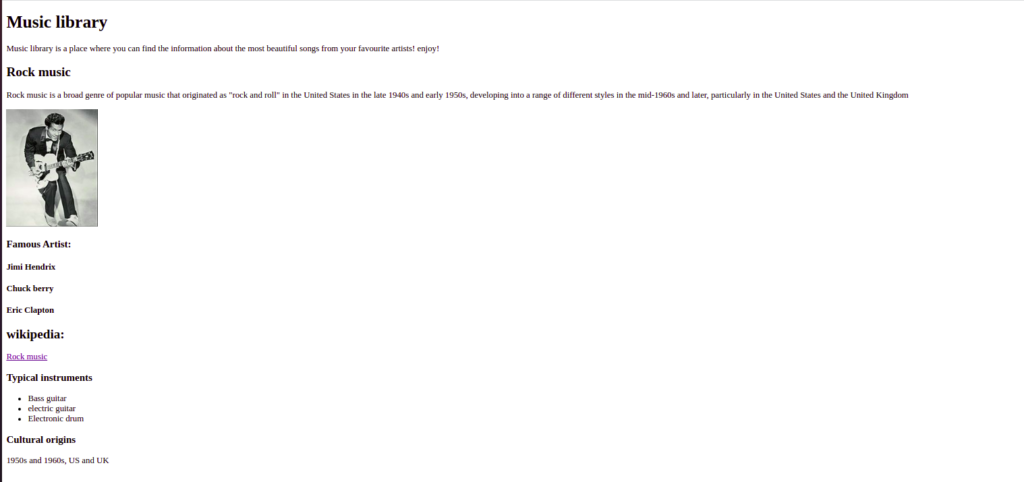
HTML template
This is the end of the Django tutorial part 2, I hope you find it useful.
I’ll see you in the next part. have fun 🙂
If you wish to join our community of self-starters learning new skills and creating projects from scratch, join our Discord channel here!
NEXT:
Django Tutorial for beginners [step by step] part 3
PREVIOUS: