Tutorial goal: Create a Django project, a simple view and return a simple response
Who this tutorial is for?
In this tutorial series, we are going to make a step-by-step tutorial about Django fundamentals. This tutorial is a complete beginner’s guide to starting learning Django. We’ve tried to make it as simple as possible. For this purpose, the concepts are divided into 10 parts. If you are a beginner, the development world may seem like an endless road, but it all depends on how to learn and practice the basics.
- For a complete guide to deploying a Django project with MySQL and Nginx using Docker see this tutorial Deploy a Django project using docker, Nginx, MySQL
Django overview
Django is a Python-based web framework that is specifically engineered to help developers build and construct powerful web applications quickly and efficiently. The core of the Django framework works with both Python versions 2 and 3. The framework was named after famous guitarist Django Reinhardt.
A framework is a toolbox of components. The reason for using a framework is to prevent written duplicate code for similar tasks. A framework is a library combined with a preset way to organize your code. It’s reusable code bundled in a reusable (but often inflexible) architecture.
Perhaps the best part about Django is its outstanding documentation. Once you learned the basics, You can use the advanced topics because Django has very thorough and readable docs available online.
Django takes much of the tedious work and repetition out of the process, solving questions such as project structure, database object-relational mapping, templating, form validation, sessions, authentication, security, cookie management, internationalization, basic administration, and an interface to access data from scripts.
Development environment
To create a Django project you need to have python installed on your machine. On Linux and MAC, it has been already installed and is ready to use. In windows, you need to install it first. Go to python.org and follow their instructions to install python on your windows machine. We will use Linux Ubuntu 18.04 but all instruction should also work on other versions of Ubuntu including 16.04 and 20.04
Create and activate virtual environment
A virtual environment, as its name implies, provides the possibility of creating a virtual and isolated environment for test and production of different projects. For example, we have two projects A and B, both of them are Django. We made A project a few years ago and started B recently. A is written with Django version 1.5 and B is made with version. We use Virtual environments so that the two projects do not overlap. This means that any changes you make to the one project environment will not affect the other projects you are developing. For example, if you install and use a specific python package and libraries in one environment doesn’t affect other environments.
Open a terminal and install virtualenv package.
sudo apt-get install virtualenv
then you need to create a virtual environment using virutalenv. We are creating a virtual environment called myenv and use python3 as the default version of python.
virtualenv myenv -p python3
Now let’s activate the virtual environment
source ./myenv/bin/activate
Notice the beginning of the line changes which shows me that my active virtual environment is (myenv).
Install Django
Now we need to install Django so that we can create our project.
pip install django
Pip utility will install the Django package and some other dependencies. Now we create our first Django project. We call the project musiclibrary
django-admin startproject musiclibrary
This command will create a folder named musiclibrary in the current directory. let’s go inside the musiclibrary folder
cd musiclibrary
Structure of a Django project
On Django, any website that is to be developed is called a “project.” A project consists of a set of “apps”. An app is also a collection of code files that are somehow separate either conceptually or functionally. If you want to make a website, the whole website will be the Django project. Likely your website will have one or more apps (for example payments, products, accounts,…) Nobody will stop you if you jam all your website functions and codes into one big app inside your project. But it is going to be painful to develop and maintain. It is like creating a house that only has one big room that has everything in it from beds to baths and kitchen and everything. It is more convenient to separate parts of the house into different rooms. In your Django projects, such structures allow developers to have an organized codebase and also reuse applications between different projects since each app is (ideally) independent of other apps.
Django follows the model – template-view (MTV) architectural pattern. The MVT (Model View Template) is a software design pattern. It is a collection of three important components:
- Model
- View
- Template
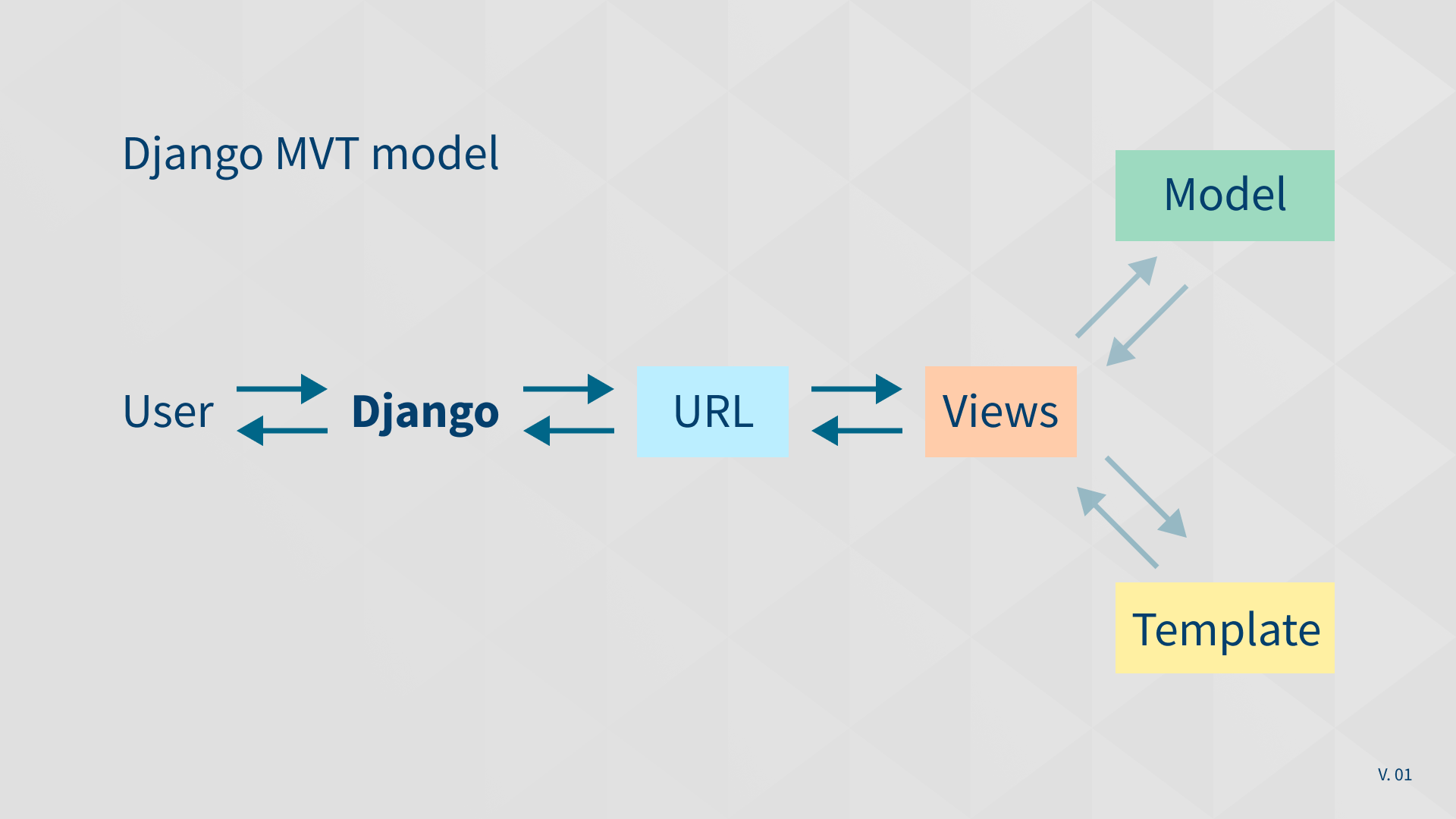
We talked about the contents of our newly created Django project. There were a manage.py file and a folder named musiclibrary. This musiclibrary folder which is inside the musiclibrary project folder (yes they have the same name) is our main app. It has been created by Django automatically and named the same as our Django project (musiclibrary). In general, a Django project consists of the following files.
musiclibrary --manage.py --musiclibrary ----__init__.py ----manage.py ----settings.py ----urls.py ----wsgi.py
The explanation of the above files is as follows:
__init__.py: A file required by Python, This file tells python to view this directory (musiclibrary folder) as python packages. Also, the above file is an empty file, and usually, you will not add anything inside it.
manage.py: One of the advantages of the command line is that it allows you to interact with this project in different ways. It allows you to do things like creating users for your website and access to the database. You should never edit or add anything to this file.
settings.py: This is a file that contains all of the Settings/configurations for your entire website. Take a look at it to see the available settings, along with the default values.
urls.py: To put it simply url.py is like a table of content for the Django website. It will direct the user requests to an appropriate view function to handle them.
wsgi.py: This file is used in deploying the project and to help your Django application communicate with the webserver. Much more on this in future sections dedicated to the deployment of Django projects.
create a view.py file
Django View is one of the essential participants of the Model View Template patterns that we discussed previously. Views act as a bridge between the MODEL and TEMPLATE, in which all the logic of the project is located. By default, views.py won’t be created when you create your project. It is expected of you to create it yourself manually. So go inside the inner musiclibrary folder (main app) and create a file and name it “views.py”. Views.py is just a simple Python file. Inside this file, you will create functions that handle user requests. Functions in views.py, take a Web request and return an HTTP response. They may require information from the models to handle the request. A view is the part of the program where the “logic” of the program is placed.
Each Django view performs a specific function and usually has an associated template file. In other words, views are responsible for interpreting user requests, transforming or fetching data associated with their requests, and passing along the proper response to the template which communicates the outcome to the user. When you visit a page on the internet or click on some links, the browser sends a request to the backend server. It contains information including headers, parameters, HTTP methods, and sometimes data.
Write our first Hello World!
Let’s create a simple hello world view to show you how views in Django work. First, we need to define our function with the def
keyword. Def
is a python registered keyword to define a function. View functions are a specific kind of function that always takes requests as an argument and they should return an HTTP response. There’s nothing special about choosing names for your functions, but it’s a good idea to name it something meaningful to make your code more legible for other developers.
In every website, each of the requests coming to the server should have a response. For example, if a user clicks on the “About” link in doprax.com, the browser makes a request and sends it to the server. There is a view (function) that handles this request and sends back a proper response to the user. This response can be HTML contents of a Web page or a redirect. It also can be a 404 error, or an XML document, or an image. Depending on the request you send different responses will send back to you. Considering this issue, a view’s only purpose is to parse requests to serve a proper response.
The example above was a very simple example of how views work. Most of the time, views do more complex things. Open the views.py file that you just created and copy-paste this little code snippet in it.
from django.shortcuts import render from django.http import HttpResponse def hello_world(request): return HttpResponse("Hello World!")
URLs in Django
URL stands for Uniform Resource Locator. As mentioned before URL is like the table of content of your website. Every page on the Internet needs its own address. It means whenever you create a view, it needs to be mapped to a URL or address. Each view is called by a URL written in the urls.py file inside the main app folder. The default urls.py file inside the main app folder looks something like this.
from django.contrib import admin from django.urls import path urlpatterns = [ path('admin/', admin.site.urls), ]
The important part of this snippet is the URL patterns, which is a python list object. Each item in this list (only one in the above example) is a mapping between an address of a page and a view function that handles it. Let’s add our first URL to the URL patterns list. It should be something like this
from django.contrib import admin from django.urls import path from .import views urlpatterns = [ path('say-hello/', views.hello_world), path('admin/', admin.site.urls), ]
Notice that, we have imported views in the third line so that we could reference the hello_world function that we have in the view.py file. We are telling Django that when a request comes to the server and the requested URL is “/say-hello/” (for example http://www.mydomain.com/say-hello/) you should call the hello_world function to handle it.
Run local development server
Let’s recap what’s happened. We installed the virtualenv package, activated it, and then installed Django using pip. We created our first Django project called musiclibrary. We created our first views function (hello_world) and mapped it to a URL (/say-hello/). Now it is time to run our project and see how it looks like in a browser. Before a request reaches our Django project it needs to be handled by a web server. Thankfully Django comes with a great built-in webserver that has everything we need out of the box. We just need to run it. Do you remember manage.py file in our project folder? Now it’s time to use it. We will run the webserver using this file.
Make sure that you have activated your virtual environment (myenv) activated. Then make sure you are at the root of the project (~/musiclibrary/). Now enter this command to start Django local development server:
python manage.py runserver
It will run the development server and by default, it will be reachable using your localhost on port 8000. Open a browser and type this URL or Just click this link (http://127.0.0.1:8000/say-hello/)
Congratulations, You just created your first Django-powered website! During this 10 part tutorial, we will gradually add more functionality to our Django website and deploy it to the cloud. At the end of this Django tutorial for beginners, hopefully, you will no longer be a beginner!
This is the end of part 1. Thank you for reading.
Click on next to continue learning.
If you wish to join our community of self-starters learning new skills and creating projects from scratch, join our Discord channel here!
NEXT: