Tutorial goal: Create a new URL, view, and template and render the model in template
Welcome to tutorial part 5. In this tutorial, we are going to show how to create a new view, model, querying in the database, and render model data in the template. This is a little bit more complex view. In the previous tutorial, you’ve learned to create a simple hello world view. But most of the time views in Django do much more complex tasks. Also, a detailed explanation about what is OuerySets and how to render data of the model in the template.
Create a view
As you know in part 1 we explained the basics about the views in Django, how to create them, and what they are doing in the Django framework. but in this section, We are going to create a more complex view because most of the time views in Django do much more complex tasks and you need to render some data into the HTML template.
let’s create a view name artist. Open the views.py module in the song app and define the artist view as follows:
def artist(request):
return HttpResponse("This is artist list")
This is a simple view that returns a simple HTTP response. let’s map a URL to this view.
from django.contrib import admin
from django.urls import path
from . import views
from song.views import artist
urlpatterns = [
path('admin/', admin.site.urls),
path('say-hello/', views.hello_world),
path('', views.home),
path('artist',artist),
]
If you run the project and click on the URL and add artists like this http://127.0.0.1:8000/artist you can see that this is a simple text that says “This is artist list”. But how about the situation that we have an artist list in the database and in case the user clicks on this URL, we want to retrieve that data.
Add some data to the admin page
before we can use Django admin to insert data manually there is a little fact you should know, it’s not usually the best way to insert data in a real-world project, obviously, it takes a significant amount of time and energy. But for now, it’s the simplest way to insert some data in the database in order to teach how to QuerySet to databases.
For inserting data manually by admin, you need to login to the admin interface that we taught in the previous section. after the login, as you can there are just 2 sections in Django admin, groups, and users:
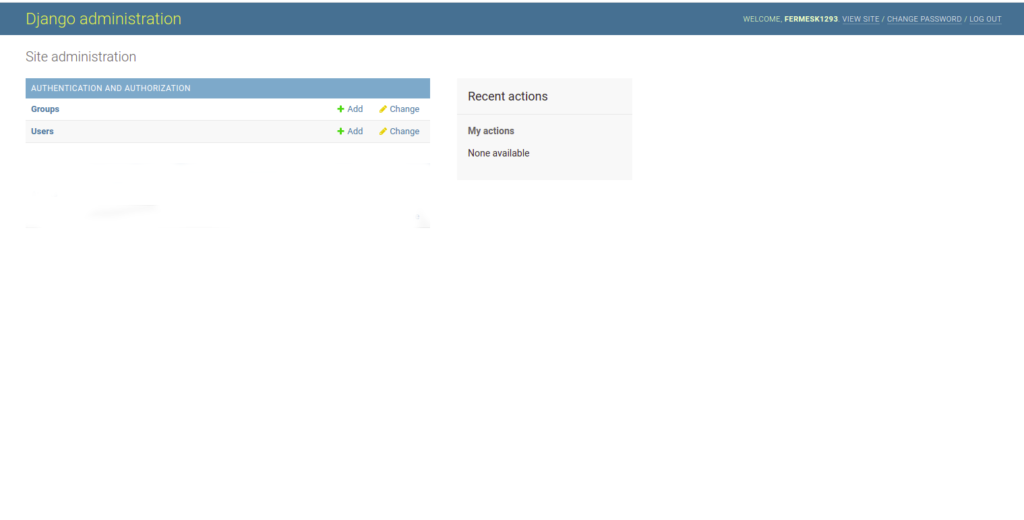
admin index page
By default, there are no models sections on the admin page. We need to tell Django to add our model(artist) section to its index page. Open admin.py module in the music app and write this code to order Django admin makes a model section:
from django.contrib import admin
from .models import Artist, Song
admin.site.register(Artist)
admin.site.register(Song)
After this, Django creates a music section with Artist model on the index page like this:
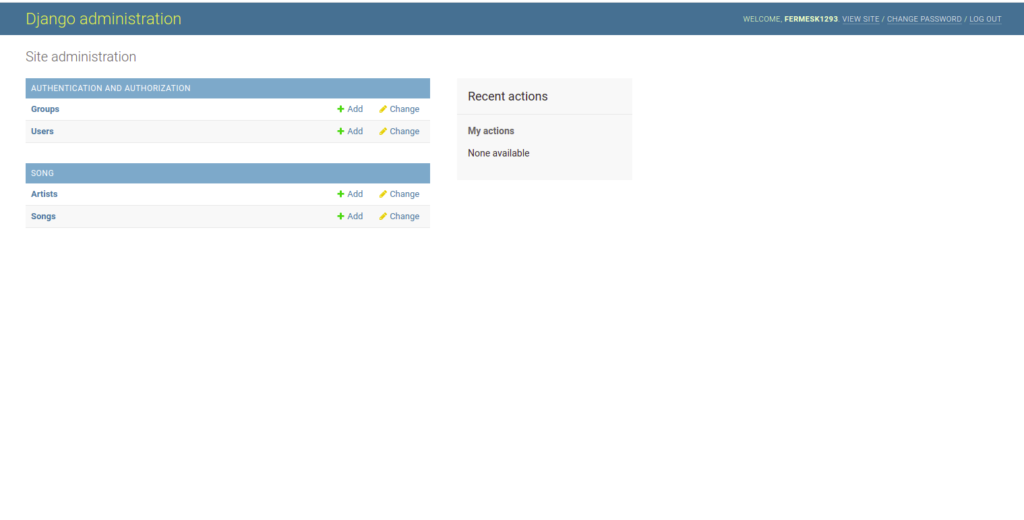
model in Django admin
Adding data to the model in Django admin
In this section, we want to add some artists to our model. Click on the add icon there will be a form, fill out this form and insert the required information like name, birth, genre. As an example we add these 4 artists:
- Kurt Cobain
- Michael Jackson
- Freddie Mercury
- Roger waters
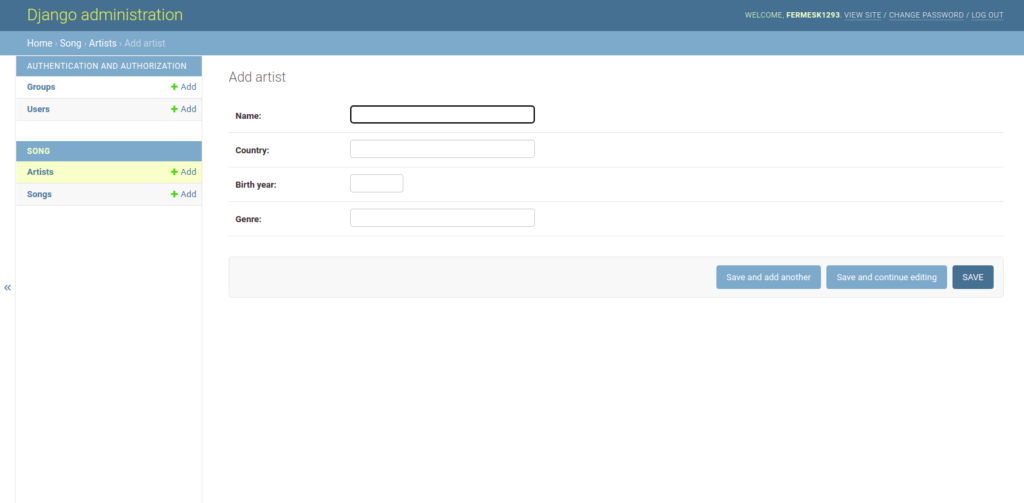
add object form
Here is a list of object artists we’ve created. Now we have 4 artists in our database.
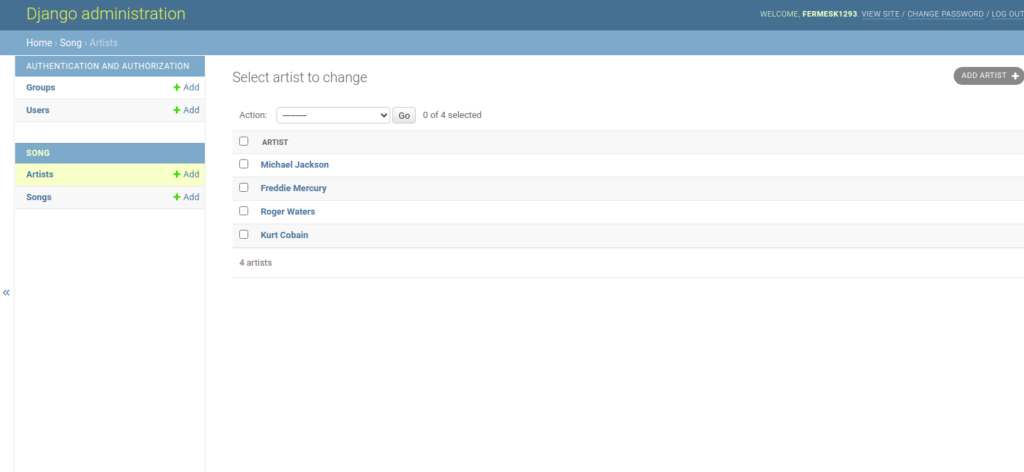
objects of the artist model in admin
We need to retrieve all objects from the database table so that we can render them within a template file. So open view.py in the music app and write this code:
def artist(request):
artist_list = Artist.objects.all()
context = { 'artist_list':artist_list }
return render(request, 'artist.html', context)
So we need to first import “render function”, in order to render the template, “artist .html”. Then import the database table(in this case Artist model) models.py file. After that pass this variable into the context dictionary so that it can be rendered out in the artist.html template page.
But usually, we need to do some changes in the template file in order to show the specific fields of the object like the name of the artist. Open the artist.html and type this code:
<!DOCTYPE html>
<html>
<body>
<h1>Artists</h1>
{%for item in artist_list%}
{{ item.name }}
{%endfor%}
</body>
</html>
And this is all that is needed to retrieve all objects (rows of data) of a database table in Django.
If you wish to join a community of self-starters learning new skills and creating projects from scratch, join our Discord channel here and share your passion.
Thank you for reading. I’ll see you in the next tutorial.