Holla chicos! Welcome to our beginner’s guide to the Spotify API! Personally, Spotify is one of my favorite websites, especially when it comes to exploring new music. Every time I discover new music, it feels like finding treasure. I’ve been eager to explore its API and create a small app. If you’re also curious about how the Spotify API works behind the scenes, you’re in the right place.
In this tutorial, we’ll take you on the basics of the Spotify API, showing you how to access its features and utilize its vast music database.
We assume that you have basic knowledge of Python. We gonna be explaining both manually and using Spotipy python package. First we start with direct way then explain Spotipy package.
What are the Spotify API use cases?
The Spotify API is really powerful, letting us access tons of info about any song or artist on Spotify. This includes details about how the music sounds, like if it’s live, acoustic, or energetic, and even info about how popular the song or artist is. We can also dig deeper and get things like where each beat falls in a song, could be very useful for advanced analysis.
Also, we can explore other awesome features of Spotify like the recommendation system and search function using the API. If you’re interested in learning more about Spotify’s Web API, you can visit their website. They even offer a console where you can test out the API’s functions, which can be really helpful if you’re having any difficulties with your own projects.
Step 1: Setting Up Your Spotify Developer Account
For start using spotify API you need to have developer account. If you don’t already have one, sign up for a Spotify account at spotify.com.
Register Your Application: Before you can authenticate with the Spotify API, you need to register your application on the Spotify Developer Dashboard. This process involves providing details about your application and receiving client credentials (Client ID and Client Secret).
For this purpose go to the Spotify Developer Dashboard and log in with your Spotify credentials. Doesn’t matter if you have a free or paid account navigate to Spotify for Developers and access your Dashboard.
For each project you can create an app and that makes it very easy to distinguish between different projects. Let’s create an app let’s name it rojyar. Rojyar in Kurdish means sun, I love sun because you know it’s warm and nice!
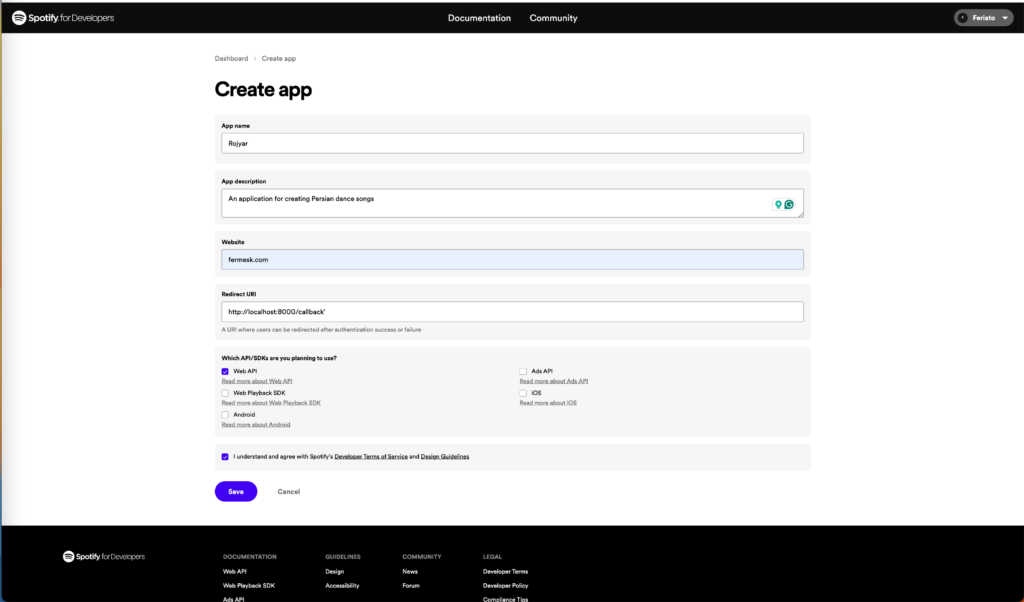
After creating the app if you click on it, you’ll see “Client ID” and “Client Secret” on the left side. These info are all we need to focus on. It’s crucial that keep credentials info secret and safe. Because if anyone gains access to them, they can easily manipulate your app, and they might do just that.
Getting authentication
Authentication with the Spotify API usually follows the OAuth 2.0 protocol, a common standard for authorization. It can be a bit tricky because you need to obtain an access token before you can explore the APIs. However, if you use the Spotipy package, it handles the token in the background, which is why we highly recommend using it to simplify the process. In this article, I’ll explain both methods briefly.
Open a Python file in vscode or any other code editor to start. I’m gonna name my file Rojyar.py. As I said earlier, I’ll first show you how authentication works without the Spotipy package. I’ll explain it with some code, and then show you how it works using Spotipy.
1)Getting authentication directly(without using Spotipy)
First thing first. Let’s import request and base64 library. In order to get access to token we need client ID and secret ID to make request to Spotify account verifying URL. Because n order to access the various endpoints of the Spotify API, we need to pass this access token. In other words if you want make request manually, this is our golden ticket to access the API.
Find client id and secret id in app setting:
import requests import json import base64 CLIENT_ID = "xxxxxxxxxxxxxxx" CLIENT_SECRET = "xxxxxxxxxxxxxxxxxx" def get_token(): auth_string = CLIENT_ID + ":" + CLIENT_SECRET auth_bytes = auth_string.encode("utf-8") auth_base64 = str(base64.b64encode(auth_bytes),"utf-8" ) url= "https://accounts.spotify.com/api/token" headers = { "Authorization": "Basic " + auth_base64, "Content-Type": "application/x-www-form-urlencoded" } data = {"grant_type": "client_credentials"} result = requests.post(url, headers=headers,data=data) json_result = json.loads(result.content) token= json_result["access_token"] return token def get_auth_token(token): return {"Authorization": "Bearer " + token} token = get_token()
What did we do here?
We designed a Python function to retrieve an access token from the Spotify API using client credentials authentication. Let’s break it down step by step:
- Importing Libraries: The code imports three libraries:
requests
,json
, andbase64
. These libraries are commonly used for making HTTP requests, handling JSON data, and encoding/decoding data in Base64 format respectively. - Setting Client ID and Client Secret: The
CLIENT_ID
andCLIENT_SECRET
variables store the client ID and client secret obtained from the Spotify Developer Dashboard. These credentials are necessary for authenticating the application with the Spotify API. - Defining the
get_token
Function: This function is responsible for obtaining an access token from the Spotify API. - Creating Authorization String: The client ID and client secret are combined into a single string separated by a colon (
:
). This string is then encoded into bytes using UTF-8 encoding. - Converting to Base64: The encoded bytes are converted into a Base64-encoded string. This string will be used for basic authentication when making the request to the Spotify API.
- Constructing the Request: The URL for requesting the access token is set to
"https://accounts.spotify.com/api/token"
. This is the endpoint provided by Spotify for obtaining access tokens. - Setting Headers: The headers for the HTTP request are defined. It includes the Authorization header with the value
"Basic "
followed by the Base64-encoded client ID and client secret, and the Content-Type header set to"application/x-www-form-urlencoded"
. - Preparing Data: The
data
dictionary contains the grant type, which in this case is"client_credentials"
. This indicates that the application is requesting an access token using only its client credentials. - Making the Request: The
requests.post
method is used to make a POST request to the Spotify API’s token endpoint. The headers and data are included in the request. - Parsing the Response: The response from the request is parsed as JSON using
json.loads()
. This gives a Python dictionary containing the response data. - Extracting the Access Token: The access token is retrieved from the JSON response dictionary using the key
"access_token"
. - Returning the Access Token: Finally, the function returns the access token obtained from the Spotify API.
We call this function whenever the application needs to access the Spotify API. Makes sense?
2)Searching for an Artist
Spotify dataset for music is amazing and the fact the API is free makes it more fascinating. There are loads of ways to get stuff from Spotify, like albums, artist details, playlists, and even special info about songs like their key or how danceable they are. To get them, we just have to ask with a special request called a GET request, and make sure we include our access_token.
This feature lets us search through Spotify’s huge music catalog, pulling up details on all sorts of songs, artists, and albums. Imagine having access to one of the world’s biggest music libraries for your projects — that’s what this API offers, opening up endless possibilities for everything from creating cool music discovery apps to building complex data analysis tools.
The Search API is flexible, letting you focus your search on specific genres, artists, or lyrics. This helps you create unique user experiences and accurate data sets. The API adds cool features like music recommendations, playlist creation, and trivia, all powered by Spotify’s huge music database. It’s easy to integrate and has helpful documentation and a strong developer community. Especially if you’re working on music-related projects.
import requests import json import base64 CLIENT_ID = "xxxxxxxxxxxxxxx" CLIENT_SECRET = "xxxxxxxxxxxxxxxxxx" def get_token(): auth_string = CLIENT_ID + ":" + CLIENT_SECRET auth_bytes = auth_string.encode("utf-8") auth_base64 = str(base64.b64encode(auth_bytes),"utf-8" ) url= "https://accounts.spotify.com/api/token" headers = { "Authorization": "Basic " + auth_base64, "Content-Type": "application/x-www-form-urlencoded" } data = {"grant_type": "client_credentials"} result = requests.post(url, headers=headers,data=data) json_result = json.loads(result.content) token= json_result["access_token"] return token def get_auth_token(token): return {"Authorization": "Bearer " + token} def search_artist(token,artist_name): url = "https://api.spotify.com/v1/search" headers = get_auth_token(token) query = f"?q={artist_name}&type=artist&limit=1" query_url = url + query result = requests.get(query_url,headers=headers) json_result = json.loads(result.content) print(json_result) token = get_token() search_artist(token,"tupac" )
What did we do here?
We searched for tupace using search api endpoint. Let’s break down our code line by line:
def get_auth_token(token):
- This line defines a function named
get_auth_token
that takes a single parameter,token
. This function is designed to prepare the authorization header needed for HTTP requests to the Spotify API.
- This line defines a function named
return {"Authorization": "Bearer " + token}
- This line within the
get_auth_token
function returns a dictionary with a single key-value pair. The key is"Authorization"
and the value is"Bearer "
concatenated with thetoken
provided. This format (“Bearer [token]”) is a standard way to include an access token in HTTP headers for OAuth 2.0 authentication.
- This line within the
def search_artist(token, artist_name):
- This line defines another function named
search_artist
, which takes two parameters:token
(the authentication token) andartist_name
(the name of the artist to search for). This function will use the Spotify Search API to find information about the specified artist.
- This line defines another function named
url = "https://api.spotify.com/v1/search"
- Inside the
search_artist
function, this line sets theurl
variable to the endpoint URL for searching within the Spotify API.
- Inside the
headers = get_auth_token(token)
- This line calls the
get_auth_token
function, passing thetoken
argument. It stores the returned dictionary (which includes the authorization header) in theheaders
variable.
- This line calls the
query = f"?q={artist_name}&type=artist&limit=1"
- This line constructs a query string for the HTTP request. It uses Python’s f-string syntax to incorporate the
artist_name
variable directly into the string. The query specifies:q
: the search query set toartist_name
.type
: the type of search, here fixed as “artist”.limit
: the number of search results to return, set to 1 for simplicity.
- This line constructs a query string for the HTTP request. It uses Python’s f-string syntax to incorporate the
query_url = url + query
- This line concatenates the base URL stored in
url
with the query stringquery
to form the full URL for the API request.
- This line concatenates the base URL stored in
result = requests.get(query_url, headers=headers)
- This line uses the
requests.get
method to send an HTTP GET request to the constructedquery_url
. It includes theheaders
dictionary, which contains the necessary authorization header. The response from the Spotify API is stored in the variableresult
.
- This line uses the
json_result = json.loads(result.content)
- This line parses the JSON content of the response (
result.content
) into a Python dictionary usingjson.loads()
, and stores it injson_result
.
- This line parses the JSON content of the response (
print(json_result)
- Finally, this line prints the dictionary
json_result
to the console, which contains the search results from the Spotify API.
- Finally, this line prints the dictionary
token = get_token()
- Outside the functions, this line assumes a function
get_token()
is defined elsewhere, which handles the retrieval of a valid OAuth token from Spotify.
- Outside the functions, this line assumes a function
search_artist(token, "tupac")
- This line calls the
search_artist
function with the retrievedtoken
and the string"tupac"
as arguments, triggering a search for the artist Tupac Shakur on Spotify.
- This line calls the
3)Output of the search api:
After running the code, Spotify sends us a JSON containing this data:
{ "artists": { "href": "https://api.spotify.com/v1/search?query=tupac&type=artist&offset=0&limit=1", "items": [ { "external_urls": { "spotify": "https://open.spotify.com/artist/1ZwdS5xdxEREPySFridCfh" }, "followers": { "href": null, "total": 17657804 }, "genres": [ "g funk", "gangster rap", "hip hop", "rap", "west coast rap" ], "href": "https://api.spotify.com/v1/artists/1ZwdS5xdxEREPySFridCfh", "id": "1ZwdS5xdxEREPySFridCfh", "images": [ { "height": 640, "url": "https://i.scdn.co/image/ab6761610000e5eb7f5cc432c9c109248ebec1ac", "width": 640 }, { "height": 320, "url": "https://i.scdn.co/image/ab676161000051747f5cc432c9c109248ebec1ac", "width": 320 }, { "height": 160, "url": "https://i.scdn.co/image/ab6761610000f1787f5cc432c9c109248ebec1ac", "width": 160 } ], "name": "2Pac", "popularity": 75, "type": "artist", "uri": "spotify:artist:1ZwdS5xdxEREPySFridCfh" } ], "limit": 1, "next": null, "offset": 0, "previous": null, "total": 1 } }
This JSON data gives information about the artist 2Pac. It provides a link to search for more artists and includes a single item representing 2Pac. You’ll find a link to his Spotify profile, and see he has over 17.6 million followers. His music spans several genres like G-Funk, Gangster Rap, Hip-Hop, and West Coast Rap. The data also includes three images of him in different sizes, his popularity score of 75 out of 100, and a unique Spotify identifier to find his music directly. This setup helps you quickly learn more about 2Pac and his style.
This was an explanation of how to get access to Spotify token and search for an artist to get the data of that artist on Spotify manually.
Spotipy package
Spotipy is a simple Python library that lets you access and interact with the Spotify Web API. With it, you can search for songs, albums, and artists, manage playlists, and get details about your favorite tracks using just a few lines of code. It makes it easy to add Spotify features to your Python projects.
My plan is to create a playlist for dancing. I love dancing, especially while working, which makes it a bit challenging to use public spaces for work :). I’m going to name it “biatooqer,” which means “Get the shaking vibe!” in Farsi. So Let’s go!
We want our playlist to include Persian dance songs. It will follow these four steps:
1)Authentication
2)Search for the songs
3)Create a playlist
4)Add songs to the playlist
1)Authentication with Spotipy
import requests import json import spotipy from spotipy.oauth2 import SpotifyOAuth CLIENT_ID = "xxxxxxxxxxxxxxxxxxxxxx" CLIENT_SECRET = "xxxxxxxxxxxxxxxxxxxx" redirect_uri='http://localhost:8000/callback' sp = spotipy.Spotify(auth_manager=SpotifyOAuth(client_id=CLIENT_ID, client_secret=CLIENT_SECRET, redirect_uri=redirect_uri, scope='playlist-modify-public' ))
As we mentioned before Spotipy is a great starting point for authentication. What we did here?
sp
: This variable is assigned the instance of spotipy.Spotify
, which will be used to interact with Spotify’s API.
SpotifyOAuth
: This is a class provided by the spotipy
library for authenticating a user via the OAuth 2.0 protocol. It helps obtain and refresh tokens needed to access specific scopes of the Spotify API.
client_id
and client_secret
: These are credentials for your application. You must obtain these from the Spotify Developer Dashboard by registering your app.
redirect_uri
: This is the URL to which Spotify will redirect after the user authorizes the application. It should match the redirect URI you’ve set in your application’s configuration in the Spotify Developer Dashboard. Check your app on dashboard to be sure it’s correct.
scope
: This defines the level of access the application will have. The scope='playlist-modify-public'
parameter requests permission to modify the public playlists of the authorized user.
2)Search for the songs
After authentication, Next step is searching for the songs that we want to add to our playlist. For this purpose, we need to specify our search criteria in our query:
query = "genre:persian year:1980-2010" results = sp.search(q=query, limit=50)
This shows how to use the spotipy
to search for music tracks based on a specific genre and release period. By leveraging the query’s targeted parameters and sp.search
, you can easily retrieve a curated list of songs that fit your desired criteria.
query
: A variable contains a search query string with specific parameters.
results = sp.search(q=query, limit=50)
Output:
The result is JSON containing data about 50 Persian dance song in this period of time. This is just a Json of a one song because outputting 50 Jsons would be a little bit too long and chaotic for this tutorial.
{"tracks": {"href": "https://api.spotify.com/v1/search?query=genre%3Apersian+year%3A1990-1999&type=track&offset=0&limit=1", "items": [{"album": {"album_type": "album", "artists": [{"external_urls": {"spotify": "https://open.spotify.com/artist/5abSRg0xN1NV3gLbuvX24M"}, "href": "https://api.spotify.com/v1/artists/5abSRg0xN1NV3gLbuvX24M", "id": "5abSRg0xN1NV3gLbuvX24M", "name": "Amr Diab", "type": "artist", "uri": "spotify:artist:5abSRg0xN1NV3gLbuvX24M"}], "available_markets": ["AR", "AU", "AT", "BE", "BO", "BR", "BG", "CA", "CL", "CO", "CR", "CY", "CZ", "DK", "DO", "DE", "EC", "EE", "SV", "FI", "FR", "GR", "GT", "HN", "HK", "HU", "IS", "IE", "IT", "LV", "LT", "LU", "MY", "MT", "MX", "NL", "NZ", "NI", "NO", "PA", "PY", "PE", "PH", "PL", "PT", "SG", "SK", "ES", "SE", "CH", "TW", "TR", "UY", "US", "GB", "AD", "LI", "MC", "ID", "JP", "TH", "VN", "RO", "IL", "ZA", "SA", "AE", "BH", "QA", "OM", "KW", "EG", "MA", "DZ", "TN", "LB", "JO", "PS", "IN", "BY", "KZ", "MD", "UA", "AL", "BA", "HR", "ME", "MK", "RS", "SI", "KR", "BD", "PK", "LK", "GH", "KE", "NG", "TZ", "UG", "AG", "AM", "BS", "BB", "BZ", "BT", "BW", "BF", "CV", "CW", "DM", "FJ", "GM", "GE", "GD", "GW", "GY", "HT", "JM", "KI", "LS", "LR", "MW", "MV", "ML", "MH", "FM", "NA", "NR", "NE", "PW", "PG", "PR", "WS", "SM", "ST", "SN", "SC", "SL", "SB", "KN", "LC", "VC", "SR", "TL", "TO", "TT", "TV", "VU", "AZ", "BN", "BI", "KH", "CM", "TD", "KM", "GQ", "SZ", "GA", "GN", "KG", "LA", "MO", "MR", "MN", "NP", "RW", "TG", "UZ", "ZW", "BJ", "MG", "MU", "MZ", "AO", "CI", "DJ", "ZM", "CD", "CG", "IQ", "LY", "TJ", "VE", "ET", "XK"], "external_urls": {"spotify": "https://open.spotify.com/album/3qZ0kZ8d3F8EpvEyx0yemA"}, "href": "https://api.spotify.com/v1/albums/3qZ0kZ8d3F8EpvEyx0yemA", "id": "3qZ0kZ8d3F8EpvEyx0yemA", "images": [{"height": 640, "url": "https://i.scdn.co/image/ab67616d0000b273d352e68d3f9ef21f6d167a96", "width": 640}, {"height": 300, "url": "https://i.scdn.co/image/ab67616d00001e02d352e68d3f9ef21f6d167a96", "width": 300}, {"height": 64, "url": "https://i.scdn.co/image/ab67616d00004851d352e68d3f9ef21f6d167a96", "width": 64}], "name": "Nour El Ein", "release_date": "1996-01-01", "release_date_precision": "day", "total_tracks": 8, "type": "album", "uri": "spotify:album:3qZ0kZ8d3F8EpvEyx0yemA"}, "artists": [{"external_urls": {"spotify": "https://open.spotify.com/artist/5abSRg0xN1NV3gLbuvX24M"}, "href": "https://api.spotify.com/v1/artists/5abSRg0xN1NV3gLbuvX24M", "id": "5abSRg0xN1NV3gLbuvX24M", "name": "Amr Diab", "type": "artist", "uri": "spotify:artist:5abSRg0xN1NV3gLbuvX24M"}], "available_markets": ["AR", "AU", "AT", "BE", "BO", "BR", "BG", "CA", "CL", "CO", "CR", "CY", "CZ", "DK", "DO", "DE", "EC", "EE", "SV", "FI", "FR", "GR", "GT", "HN", "HK", "HU", "IS", "IE", "IT", "LV", "LT", "LU", "MY", "MT", "MX", "NL", "NZ", "NI", "NO", "PA", "PY", "PE", "PH", "PL", "PT", "SG", "SK", "ES", "SE", "CH", "TW", "TR", "UY", "US", "GB", "AD", "LI", "MC", "ID", "JP", "TH", "VN", "RO", "IL", "ZA", "SA", "AE", "BH", "QA", "OM", "KW", "EG", "MA", "DZ", "TN", "LB", "JO", "PS", "IN", "BY", "KZ", "MD", "UA", "AL", "BA", "HR", "ME", "MK", "RS", "SI", "KR", "BD", "PK", "LK", "GH", "KE", "NG", "TZ", "UG", "AG", "AM", "BS", "BB", "BZ", "BT", "BW", "BF", "CV", "CW", "DM", "FJ", "GM", "GE", "GD", "GW", "GY", "HT", "JM", "KI", "LS", "LR", "MW", "MV", "ML", "MH", "FM", "NA", "NR", "NE", "PW", "PG", "PR", "WS", "SM", "ST", "SN", "SC", "SL", "SB", "KN", "LC", "VC", "SR", "TL", "TO", "TT", "TV", "VU", "AZ", "BN", "BI", "KH", "CM", "TD", "KM", "GQ", "SZ", "GA", "GN", "KG", "LA", "MO", "MR", "MN", "NP", "RW", "TG", "UZ", "ZW", "BJ", "MG", "MU", "MZ", "AO", "CI", "DJ", "ZM", "CD", "CG", "IQ", "LY", "TJ", "VE", "ET", "XK"], "disc_number": 1, "duration_ms": 307762, "explicit": false, "external_ids": {"isrc": "EGA020501535"}, "external_urls": {"spotify": "https://open.spotify.com/track/3ZTuYuaV1fhdNnuIBuzTYy"}, "href": "https://api.spotify.com/v1/tracks/3ZTuYuaV1fhdNnuIBuzTYy", "id": "3ZTuYuaV1fhdNnuIBuzTYy", "is_local": false, "name": "Nour El Ein", "popularity": 63, "preview_url": "https://p.scdn.co/mp3-preview/9e1a494d69c5ed15080af07b5b948a274da7b730?cid=38c1f30cceb047bea69b89f1c5f04863", "track_number": 1, "type": "track", "uri": "spotify:track:3ZTuYuaV1fhdNnuIBuzTYy"}], "limit": 1, "next": "https://api.spotify.com/v1/search?query=genre%3Apersian+year%3A1990-1999&type=track&offset=1&limit=1", "offset": 0, "previous": null, "total": 100}}
3)Create a playlist
In this part we created a playlist on Spotify.
playlist_name = "biatouqer" playlist_description = "A playlist of Persian pop songs for dancing while working!" playlist = sp.user_playlist_create(sp.me()['id'], playlist_name, public=True, description=playlist_description) track_titles = [track['name'] for track in results['tracks']['items']] def get_track_id(title): result = sp.search(q=title, limit=20) if result['tracks']['items']: return result['tracks']['items'][0]['id'] else: print(f"No track found for title: {title}") return None
What did we do here? We:
- 1)Create a new playlist with a specific name and description.
- 2)Extract track titles from a search query.
- 3)Use the helper function to find track IDs based on their titles and add them to your newly created playlist.
- Also Here’s a detailed explanation of the various components:
- Creating the Playlist:
playlist_name
: This is the name of playlist.You can call it whatever you want.playlist_description
: A brief description for the playlist, indicating that it’s a collection of Persian pop songs for dancing while working. writing better description makes your playlist stand out in Spotify.sp.user_playlist_create
: This function creates a new playlist in the authorized user’s Spotify account.sp.me()['id']
: Retrieves the authenticated user’s Spotify ID.public=True
: Makes the playlist publicly accessible.description=playlist_description
: Adds a description to the playlist.
playlist
variable. - Extracting Track Titles:
track_titles
: A list comprehension that extracts the names of all tracks found in the search results (results['tracks']['items']
).- This will provide a list of titles that you can later use for further processing.
- Defining a Helper Function to Retrieve Track IDs:
get_track_id
: A function that accepts a track title as an argument and searches Spotify’s library for matching tracks.sp.search
: Executes a search query using the given title with a limit of 20 tracks.result['tracks']['items']
: If there are matching tracks, the function returns the ID of the first one.- If no matching track is found, a message is printed, and
None
is returned.
When you run this part of code, Spotify asks you to authorized the app with this msg:
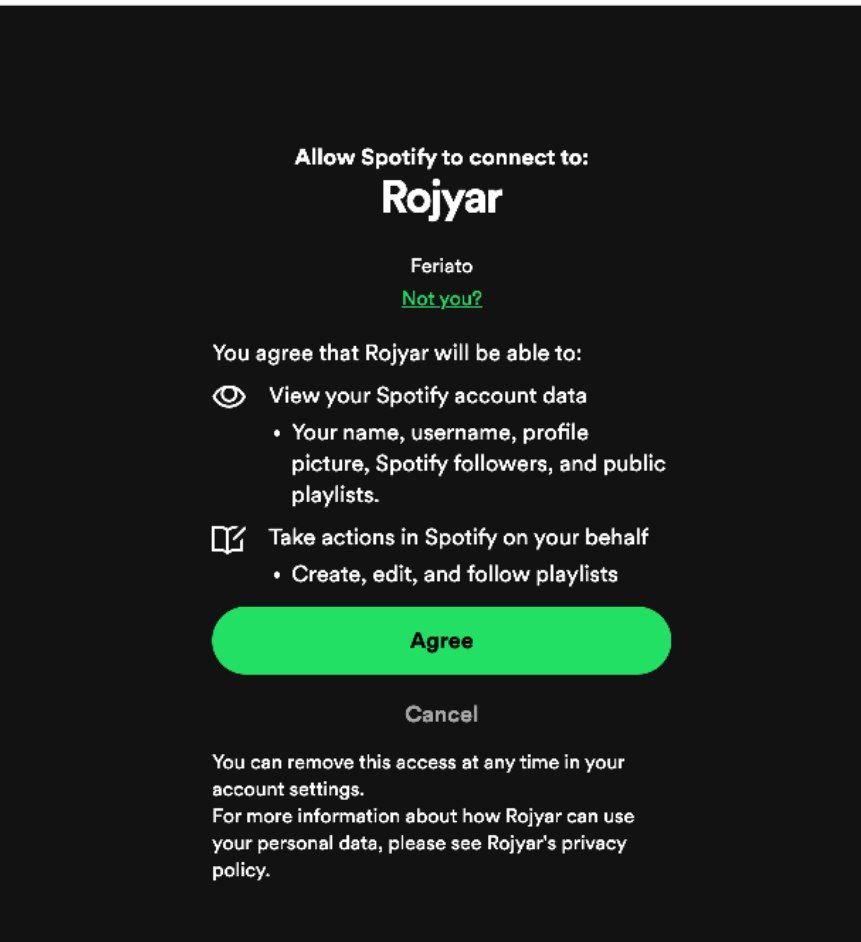
4)Adding songs to the playlist
And at this part of code, we add songs to the playlist based on their titles:
for title in track_titles: track_id = get_track_id(title) if track_id: sp.playlist_add_items(playlist['id'], [track_id]) print("Tracks added to the playlist successfully!")
As you can see It’s an easy and super fast way to organize and customize your playlist with your favorite tracks. Here is detailed explanation of what we did:
Loop Through Track Titles:
The for
loop iterates through each track title in the track_titles
list.
Get Track ID:
For each track title, the get_track_id
function is called to search Spotify’s database and obtain the corresponding track’s unique Spotify ID.Check for Valid Track ID:
- If the
get_track_id
function returns a valid ID (meaning that a track was found for the given title), it proceeds to add this track to the playlist. - If no track was found (indicated by the function returning
None
), the loop skips to the next title.
Add Track to Playlist:
sp.playlist_add_items
:- This method adds the track to the playlist using its Spotify ID.
playlist['id']
: The unique identifier of the newly created playlist.[track_id]
: The ID of the track to be added is wrapped in a list since this method expects a list of track IDs.
Here we go! We’ve created our playlist. This is the link on Spotify:
I hope you enjoy my tutorial and find it helpful, if you have any questions feel free to reach out. Cheers ?