Django Tutorial for Beginners [Step by Step] – Part 8: User Authentication in Django
Welcome to Part 8 of our Django tutorial series.
In this tutorial, we will build a sign-up form and explore how user authentication works in Django. Users will be able to register on our website, and we will configure permissions to allow them to log in.
Since almost every web project requires a sign-up and login system, this tutorial will cover the core steps involved, including creating a new app, defining views and URLs, and using Django’s built-in authentication system.
Prerequisites
- Knowledge of creating a basic Django project
- Knowledge of Django's Model-View-Template (MVT) architectural pattern
Refer to parts 1–7 of this tutorial series to get a basic understanding of the above.
Overview of user authentication in Django
Django provides a built-in user authentication system that handles both authentication (verifying a user’s identity) and authorization (determining what an authenticated user is allowed to do).
By default, the required configuration is already included in the settings.py to make it easier to integrate user management features.
Creating the sign-up app
To keep our project structured, we will create a separate Django app for managing user authentication (sign up and sign in). This approach ensures that everything related to user sign-up and sign-in is organized in separate pages to make it easy for us in the future to manage our project's users and subjects related to user sign-up and sign-in.
Run the following command to create a new app named account:
python manage.py startapp account
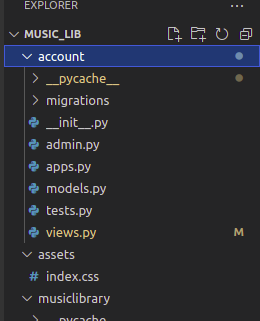
Setting up the sign-up URL and view
Next, we need to define the URL for our sign-up page. Open the urls.py file in the main Django app and add the following entry:
path('signup/',signup),
Now, let’s define the signup view inside views.py in the account app. We'll first import some necessary packages and create a function called signup in views.py and an HTML file in the Templates directory, like this:
from django.shortcuts import render, redirect, reverse
from django.contrib.auth import login, authenticate
from django.contrib.auth.forms import UserCreationForm
from django.contrib.auth.decorators import login_required
def signup(request):
return render(request,'signup.html')
Then, head over to the templates directory and create a new HTML file and name it signup.html, then input the line of code:
touch signup.html
At this point, if you visit http://127.0.0.1:8000/signup/, you will see an empty page because we haven’t created the HTML form yet.
Creating the sign-up form
Django provides a built-in UserCreationForm, which simplifies user registration by including fields for username, password, and password confirmation.
One of the advantages of this form Django provides is that it comes with frontend code so there is no need to write frontend code. It also includes validation that checks if the username already exists or not.
Update the signup view to handle user registration:
def signup(request):
if request.method == 'POST':
form = UserCreationForm(request.POST)
if form.is_valid():
form.save()
return redirect('home')
else:
form = UserCreationForm()
return render(request, 'signup.html', {'form': form})
How it works:
- If the request method is POST, the form is submitted with user data.
- The form is validated, and if valid, the new user is saved in the database.
- The login(request, user) function automatically logs in the user after sign-up.
- Finally, the user is redirected to the home page.
Now, go ahead and create an HTML template to display the form. Inside the templates directory, create a file called signup.html and add the code for the frontend form.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sign Up</title>
</head>
<body>
<h2>Sign Up</h2>
<form method="POST">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Sign Up</button>
</form>
</body>
</html>
Don’t forget to add the name as a third parameter to the home URL in urls.py as follows; otherwise, you will get an error.
path('', views.home,name="home"),
This means after the user signs up, it will redirect them to the home page.
If you go to 127.0.0.1:8000/signup/, you should see a page like this:
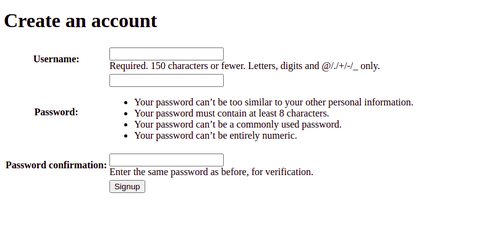
Understanding HTTP Methods: GET vs POST
In web development, we commonly use two HTTP request methods to either send data or retrieve data from the backend or server:
- GET: Used to retrieve data from the server. For example, when you type a URL in the address bar, it is a GET request because you request to get an HTML file from the server. (e.g., when you visit signup/, Django sends the signup form)
- POST: Used to send data to the server. For example to to add some records to the server. (e.g., when you submit the sign-up form).
The GET and POST requests can both go to the same URL so we can send get request to
"/signup" to receive an HTML file from the server. We can also send a POST request from the same page to post data.
In our case:
- GET request → Displays the sign-up form.
- POST request → Submits the user data for registration through the sign-up form.
Django’s request.method helps us handle both cases inside the signup view.
In practice these days, it’s more unlikely to use this user creation form, but in general, while learning Django, it’s nice to get familiar with it.
What we did in the sign-up code was to redirect the user after the signup. In most cases, we'd want the user to log in to the application after signing up instead of directing them to the home page.
For this purpose, we need to add a login function and store f.save() into a variable, and pass this variable to the login function in the signup view. open views.py:
def signup(request):
if request.method == 'POST':
form = UserCreationForm(request.POST)
if form.is_valid():
user = form.save()
login(request,user)
return redirect('home')
else:
form = UserCreationForm()
return render(request, 'signup.html', {'form': form})
So if the form is valid, the form would be saved in a variable (in our case, user), and then we pass this user to the login function in the next line.
This is how we create a user creation form in Django.
Conclusion
In this tutorial, we learned how to:
- Set up a sign-up system in Django.
- Use Django’s built-in authentication system for user registration.
- Handle GET and POST requests in Django views.
- Use Django’s UserCreationForm for quick user authentication.
In the next tutorial (Part 9), we will learn how to log users in after successful registration.
Next:
Django Tutorial for Beginners [Step by Step] - Part 9: User Login and Authorization.
If you wish to join a community of self-starters learning new skills and creating projects from scratch, join our Discord channel and share your passion.