Django Tutorial for Beginners [Step by Step] – Part 3: Virtual Environment and New App
Tutorial goal: Access the shell, set up a virtual environment, create a new app, and install it.
In this tutorial, we will add a new app to our Django project.
Prerequisites
- Knowledge of creating a basic Django project
- Knowledge of Django's Model-View-Template (MVT) architectural pattern, view, and Django template
Refer to parts 1 and 2 of this tutorial series to get a basic understanding of the above.
Understanding Django Apps
Before we get started creating a new app, you need to understand the difference between a project and an app in Django.
Imagine a large company with multiple departments. The company itself represents a Django project, while each department is analogous to a Django app.
Each department has it’s own facilities and functionalities and each focuses on a specific set of problems, but all work together for a common goal. Similarly, a Django project can contain multiple apps, each handling a distinct part of the project.
For example:
- An account app might handle user registration, login, password recovery, and other account-related features.
- A payment app could manage payments, invoices, and coupons.
There are several benefits to using multiple apps:
- First, this makes it easy for you to organize your code. You can structure your code more effectively by separating concerns into distinct apps.
- You can reuse your code across multiple projects. For example, some parts of a payment app developed for one website could easily be used in another project.
Creating a New App in Django
Creating a new app in Django is simple. Here’s how you do it:
- Make sure your virtual environment is activated before proceeding. This ensures that any dependencies or packages are installed in the correct environment.
- Use the startapp command to create a new app. It’s important to name the app something meaningful so that it clearly represents its function within the project and makes it easy for other developers to know its use at a glance.
Run the following command:
python manage.py startapp song
As mentioned in previous tutorials, manage.py is a crucial file located at the root of your Django project. It’s used to execute a variety of Django-specific tasks, such as creating a new app, running the development server, executing tests, and more.
After running the command above, a folder named after your app (e.g., song) will be created within the project directory. At this point, your project folder structure will look something like this:
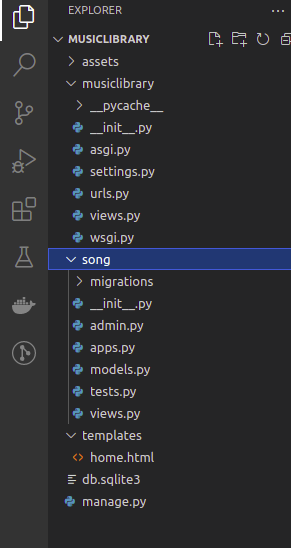
By creating (starting) a new Django app, it will not be picked up by Django automatically, unless we tell Django that we have a new app and it should be installed.
This kind of configuration (settings) is done in the settings.py file in the main app folder. So open your settings.py file and let’s install our newly created app.
Find the section of installed apps, which is a list named INSTALLED_APPS. It should look like this by default:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
]
Django by default has some pre-installed apps that are provided for developers’ convenience. If you open settings.py in the project directory, you can see this list of preinstalled apps.
You can install a Django app by providing its name to the list of INSTALLED_APPS in settings.py.
Now we add the name of our new app 'song' to this list.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'song',
]
And that's all you need to know about creating a new app in your Django project.
Thank you for reading. I’ll see you in the next tutorial.
If you wish to join a community of self-starters learning new skills and creating projects from scratch, join our Discord channel and share your passion.
Next:
Django Tutorial for Beginners [Step by Step] – Part 4: Model and Database in Django.