Django Tutorial for Beginners [Step by Step] – Part 1: Creating a Django Project & Building a Simple View
In this tutorial, you will learn how to create a Django project, set up a simple view, and return a basic response.
Prerequisites
To start with Django, you need Python installed on your machine.
- Linux & macOS: Python is pre-installed and ready to use.
- Windows: Download and install Python from python.org by following the installation guide.
For this tutorial, we will use Ubuntu 18.04, but the instructions will also work for Ubuntu 16.04, 20.04, and other versions.
Who is this tutorial for?
This step-by-step guide is designed for absolute beginners who want to learn Django from the ground up. The concepts are broken down into 10 parts to make learning easier. If you're new to web development, it may seem overwhelming at first, but mastering the basics through practice will set you on the right path.
For an advanced guide on deploying a Django project with MySQL and Nginx using Docker, check out our article on: Deploying a Django Project with Docker, Nginx, and MySQL
What Is Django?
Django is a Python-based web framework designed to help developers build powerful web applications quickly and efficiently. It supports both Python 2 and 3 and is named after the legendary jazz guitarist Django Reinhardt.
A framework is essentially a collection or toolbox of pre-built components that help streamline development by preventing repetitive coding for similar tasks. It's like reusable code bundled in a reusable (but often inflexible) architecture.
Django provides an organized structure, making it easier to build scalable applications with built-in tools for:
- Database management (ORM)
- Templating
- Form validation
- Session handling
- Authentication & security
- Internationalization
- Admin panel & API access
One of Django’s biggest strengths is its comprehensive documentation, which makes it easy to learn and explore advanced topics as you progress.
Now, let's get started with creating a Django project.
Create and activate virtual environment
First, what is a virtual environment?
A virtual environment is an isolated space where you can install and manage packages separately for different projects. This allows you to work on multiple projects without conflicts between their dependencies.
With a virtual environment, you are able to create a virtual and isolated environment for testing and production of different projects.
For example:
- Project A (created years ago) uses Django 1.5.
- Project B (a new project) uses a newer Django version.
Without a virtual environment, managing these two projects on the same system could cause version conflicts. By using virtual environments, any changes or package installations in one project will not affect other projects.
For example, if you install and use a specific Python package and libraries in one environment, it doesn’t affect other environments.
This helps keep your development environment clean and organized.
To get started, open a terminal and install the virtualenv package using this command:
sudo apt-get install virtualenv
Next, create a virtual environment using virtualenv. In this example, we will name it "myenv" and set Python 3 as the default version:
virtualenv myenv -p python3
Once created, activate the virtual environment with:
source ./myenv/bin/activate
After activation, you’ll notice that your terminal prompt changes to (myenv), indicating that the virtual environment is now active.
Installing Django and Creating a Project
First, install Django using pip:
pip install django
This will install Django along with its required dependencies.
Creating Your First Django Project
Now, let’s create a new Django project called musiclibrary:
django-admin startproject musiclibrary
This command creates a directory named musiclibrary containing the necessary project files. Navigate into the project folder:
cd musiclibrary
Understanding Django Project Structure
On Django, any website that is to be developed is called a project. And a project is a collection of apps. A Django app is a self-contained module that handles a specific functionality, such as authentication, payments, or blog posts.
For example, if you build an e-commerce website, the project is the entire website, while different functionalities (e.g., payments, products, accounts) are separate apps. Keeping apps modular makes your codebase more organized and reusable.
Django follows the Model-View-Template (MVT) architectural pattern, which consists of:
- Model: Defines the structure and behavior of the database (e.g., tables, fields, relationships).
- View: Handles the logic, processes user requests, and interacts with the model.
- Template: Defines the front-end structure (HTML files) used to render data dynamically.
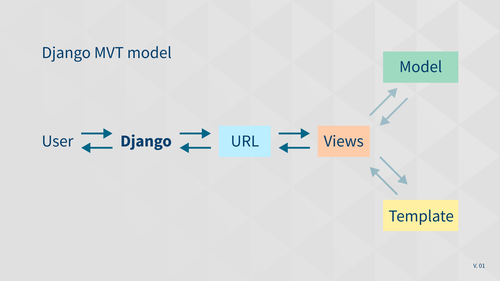
This separation makes Django applications well-structured, scalable, and easy to maintain.
When you create a new Django project, it generates a set of essential files and directories.
musiclibrary
--manage.py
--musiclibrary
----__init__.py
----manage.py
----settings.py
----urls.py
----wsgi.py
Let's explore each of them:
- manage.py: A command-line utility that helps manage your Django project. You use it to run the development server, apply migrations, create apps, and more. You should never edit or add anything to this file.
- musiclibrary/ (Inner Directory): This is the core of your Django project. It shares the same name as your project but serves a different role. It contains:
- __init__.py: A file required by Python. This file tells python to view this directory (musiclibrary folder) as a Python package. This file is an empty file, and usually you will not add anything inside it.
- settings.py: Contains all configurations for your project, including database settings, installed apps, middleware, and static files.
- urls.py: Defines URL routing for your project. It is like a table of content for the Django website. It will direct the user requests to an appropriate view function to handle them.
- wsgi.py: The entry point for WSGI (Web Server Gateway Interface) applications, used for deploying Django projects and to help your Django application communicate with the webserver. We'll discuss more on this in future sections dedicated to the deployment of Django projects.
This structure forms the foundation of every Django project and provides the necessary components to build and scale web applications effectively.
Create a view.py file
In Django’s Model-View-Template (MVT) architecture, the View serves as the link between the Model (database) and the Template (HTML UI). It contains the logic that determines how data is processed and presented to users.
What is a Django view?
A Django view is a Python function (or class) that receives a web request and returns an HTTP response. The response could be:
- Raw data (e.g., JSON, XML)
- An HTML template with dynamic content
- A redirect to another URL
By default, Django does not generate a views.py file when you create a new project. You must manually create it inside your main app’s directory.
Navigate to your inner musiclibrary folder (the main app) and create a file named views.py. Inside this file, you will create functions that handle user requests. Functions in views.py take a web request and return an HTTP response. They may require information from the models to handle the request.
How views work in Django
- A user visits a webpage.
- The browser sends a request to Django’s backend.
- Django routes the request to the correct view function based on URL patterns.
- The view processes the request, fetches or manipulates data, and returns an HTTP response.
- If needed, the response is passed to a template, which renders HTML before sending it back to the user.
Views are the core logic layer of Django applications that handles data retrieval, processing, and generating of responses.
Write our first Hello World!
To understand how views work in Django, let's start by creating a simple "Hello, World!" view.
Every request made to a Django application is handled by a specific view that determines the response.
For example, when a user clicks on the "About" link in doprax.com, the browser sends a request to the server. Django's URL dispatcher directs this request to the correct view function, which then returns an appropriate response. This response could be an HTML webpage, a redirect to another URL, or even a 404 error page.
To define a function, we need to use the def keyword. Def is a Python-registered keyword for defining functions.
You don't have to put too much thought into choosing a name for your function, but it’s always a good idea to name it something meaningful to make your code more legible for other developers.
Writing your first Django view ("Hello, World!")
Open the views.py file in your main app (musiclibrary), and add the following code:
from django.shortcuts import render
from django.http import HttpResponse
def hello_world(request):
return HttpResponse("Hello World!")
URLs in Django
URL stands for Uniform Resource Locator. As mentioned before, the URL is like the table of contents of your website. Every page on the Internet needs its own address. It means whenever you create a view, it needs to be mapped to a URL or address. Each view is called by a URL written in the urls.py file inside the main app folder. The default urls.py file inside the main app folder looks something like this.
from django.contrib import admin
from django.urls import path
urlpatterns = [
path('admin/', admin.site.urls),
]
The important part of this snippet is the URL pattern, which is a Python list object. Each item in this list (only one in the above example) is a mapping between an address of a page and a view function that handles it.
Let’s add our first URL to the URL patterns list. It should be something like this:
from django.contrib import admin
from django.urls import path
from .import views
urlpatterns = [
path('say-hello/', views.hello_world),
path('admin/', admin.site.urls),
]
Notice that we have imported views in the third line so that we could reference the hello_world function that we have in the view.py file. We are telling Django that when a request comes to the server and the requested URL is “/say-hello/” (for example, http://www.mydomain.com/say-hello/), you should call the hello_world function to handle it.
Run local development server
Let's review what we've done so far:
- Installed virtualenv, created, and activated a virtual environment.
- Installed Django using pip.
- Created our first Django project called musiclibrary.
- Defined our first view function (hello_world) and mapped it to a URL (/say-hello/).
Now, it's time to run our project and view it in a browser.
Before a request reaches our Django project, it needs to be handled by a web server. Thankfully, Django comes with a built-in development server, which provides everything we need for local testing.
Remember the manage.py file in our project folder? Now it’s time to use it.
Make sure that your virtual environment (myenv) is activated. Then make sure you are at the root of the project (cd ~/musiclibrary/).
Now enter this command to start the Django local development server:
python manage.py runserver
It will run the development server and by default, it will be reachable using your localhost on port 8000. Open a browser and type this URL or Just click this link (http://127.0.0.1:8000/say-hello/)
Congratulations. You just created your first Django-powered website!
This is the end of Part 1.
During this 10-part tutorial, we will gradually add more functionality to our Django website and deploy it to the cloud. At the end of this Django tutorial for beginners, hopefully, you will move from being a beginner to being an intermediate Django user.
Click on the next tutorial to continue learning.
If you wish to join our community of self-starters learning new skills and creating projects from scratch, join our Discord channel.
Next:
Django Tutorial for Beginners [Step by Step] – Part 2: Django Template.