Power to run your {code} 🚀
Power your app development with scalable virtual machines and a vibrant app market. Build, share, and connect like never before.
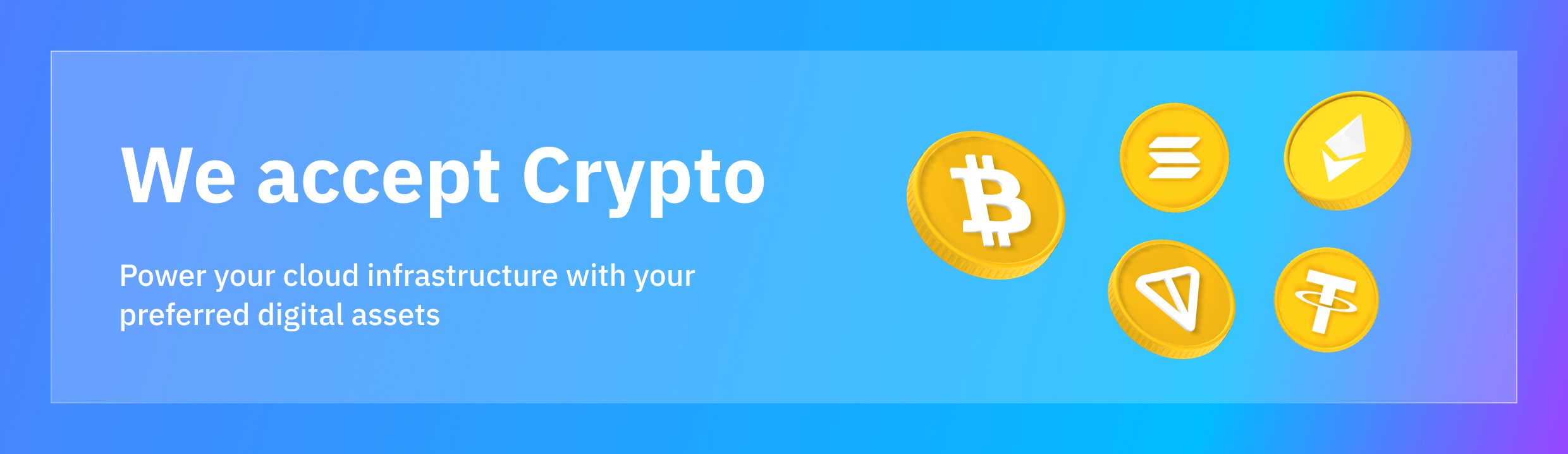
Why Choose Doprax?
Everything you need to innovate, collaborate, and succeed—on one platform.
Global Presence for Innovation 🌎
Operate with speed and reliability, no matter where you are. Doprax offers coverage in 40 locations across 20 countries, ensuring optimal performance for developers worldwide.
40 locations strategically distributed for low latency.
Global accessibility and local compliance.
Seamless scalability no matter your region.
Your Favorite Tools & Databases, Fully Supported 🛠️
Doprax integrates with a wide range of tools and databases to enhance your development experience.
MongoDB
PostgreSQL
MySQL
Neo4j

Logstash
MariaDB
Redis

Kibana
Elasticsearch
Fast network ⚡️, Secure Connections 🔒️
Doprax provides a fast and secure networking infrastructure to ensure seamless connectivity and top-tier performance.Ideal for VPN networks and distributed systems.
VPN Servers
Network tunnels
100% secure
Build Anything You Can Imagine
Doprax provides the flexibility and tools to create a wide variety of applications
CMS
Platform supports CMS deployment easily and scalable
VPN Servers
Quickly spin up a VPN server using V2ray, OpenVPN, XUI, and more. One click installation
Databases
Mysql, Postgres, Elasticsearch Mongodb, …
APIs
Create and deploy your backend APIs for your web or mobile app in your favorite programming language
Static websites
Get all the benefits of static code. Quickly build a superfast and stable static website on Doprax Cloud.
Virtual machines
Run and manage virtual machines with ease. Deploy apps to your virtual machines in a few clicks
Affordable Plans for Everyone
Choose the plan that fits your needs, whether you’re experimenting or scaling globally.
Container platrorm
From
$3.95
/month
All the basic features to boost your deployment.
1 – 8 GB RAM
1 – 4 vCPU cores
1 – 8 GB RAM
1 – 8 GB RAM
1 – 8 GB RAM
Virtual Machines
From
$5.95
/month
All the basic features plus more to boost your deployment
2 – 8 GB RAM
1 – 4 vCPU cores
20 – 160 GB SSD
1 dedicated IPv4 address
1 dedicated IPv6 address range
20 TB of traffic / month
Live Chat Support
Enterprise App
For pricing
Contact sales
All the basic features plus extra more to boost your deployment
Custom solution
Dedicated hardware resources
Custom SLA
Priority Support
Got questions? We’ve got answers.
From getting started to mastering advanced features, here’s everything you need to know about Doprax. If you’re still curious, our support team is just a click away!
What payment method do you accept?
We offer a variety of convenient payment methods to suit your needs. We accept:
1.Major Credit Cards: Visa, Mastercard, etc.
2.WeChat Pay: For users in China (我们接受微信支付,适用于中国用户).
3.Zarinpal: For users in Iran (پرداخت با زرین پال).
4.Cryptocurrencies: Over 300 accepted, including Bitcoin, Ethereum, and many more.
Will you help transfer from my current web host?
We offer free migration assistance! Sign up with a credit card and contact support@doprax.com to get started.
Are Doprax virtual machines billed per hour?
Yes! Absolutely! In Doprax you only pay the server costs per hours.
How many cloud providers and locations does Doprax support?
Doprax supports 4 major cloud providers (Hetzner, DO, Vultr, and OVH) with a total of 40 global locations and counting for your deployments.
Need more help?
Contact support